In this article, we will dive into how to build a chatbot using Python, focusing on advanced features such as context handling, real-time data integration, and machine learning. This guide is designed for those who want to take their chatbot development to the next level, creating more human-like interactions.
Key Features of an Advanced Chatbot
- Contextual Understanding: The chatbot can remember previous interactions and manage multi-turn conversations.
- Natural Language Processing (NLP): Implement deep learning techniques to analyze and respond with context-aware, meaningful replies.
- API Integration: The bot can pull live data (like weather or stock prices) from external APIs to provide real-time information.
- Machine Learning: Use learning algorithms to improve the bot’s conversational abilities over time.
Step-by-Step Guide to Build an Advanced Chatbot
1. Set Up the Development Environment
First, ensure that Python and necessary libraries are installed. Run the following commands:
pip install transformers tensorflow nltk spacy
These libraries will be the foundation of the chatbot’s language processing and deep learning capabilities:
- Transformers for NLP models.
- TensorFlow for deep learning.
- NLTK and SpaCy for text processing.
2. Pretrained Model for Natural Language Understanding
You can utilize Hugging Face’s DialoGPT for generating human-like responses. Here’s a simple example of how to use it:
from transformers import pipeline
# Load the conversational model
chatbot_pipeline = pipeline('conversational', model="microsoft/DialoGPT-large")
# Generate a response
user_input = "What's the weather today?"
response = chatbot_pipeline(user_input)
print(response)
3. Implement Contextual Memory
For multi-turn conversations, the chatbot needs to remember previous inputs. You can use conversational models to store dialogue history:
from transformers import Conversation, pipeline
chatbot_pipeline = pipeline('conversational', model="microsoft/DialoGPT-large")
# Initialize conversation object
conversation = Conversation()
# Add input and generate response
conversation.add_user_input("Tell me a joke.")
response = chatbot_pipeline(conversation)
print(response.generated_responses[-1])
This allows the chatbot to maintain context throughout the conversation.
4. Real-Time API Integration
To provide real-time responses (e.g., weather updates), integrate external APIs. Here’s how to build a chatbot with simple weather API integration:
import requests
def get_weather(city):
api_key = 'your_openweather_api_key'
base_url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(base_url).json()
if response['cod'] == 200:
main = response['main']
temp = main['temp'] - 273.15 # Convert from Kelvin to Celsius
return f"The current temperature in {city} is {temp:.2f}°C."
else:
return "City not found."
print(get_weather("New York"))
By integrating APIs, your chatbot can provide real-time information on various topics like weather, stock prices, or news.
5. Machine Learning for Self-Learning
To build a truly advanced chatbot, implementing reinforcement learning or supervised learning can allow your bot to learn from user interactions.
This can be done by gathering user input and feedback, creating a dataset, and retraining your chatbot periodically.
Using TensorFlow or PyTorch, you can fine-tune models to enhance your bot’s language comprehension and response quality.
Enhancements for an Advanced Chatbot
- Voice Input/Output: Incorporate Google’s SpeechRecognition and pyttsx3 libraries for speech-to-text and text-to-speech.
- Sentiment Analysis: Using libraries like NLTK, your chatbot can adjust its tone based on the user’s sentiment. For example, a negative sentiment could trigger an empathetic response.
from nltk.sentiment import SentimentIntensityAnalyzer
sia = SentimentIntensityAnalyzer()
user_input = "I'm feeling sad."
sentiment = sia.polarity_scores(user_input)
print(sentiment)
- Deployment: You can deploy your chatbot on messaging platforms like Telegram, Slack, or even a website using Flask or Django.
Internal and External Links
- Internal link: How to Build a Chatbot in Python (intermediate)
- External link: Hugging Face’s Transformers Documentation
Conclusion
To build a chatbot in Python requires integrating various technologies like NLP (Natural Language Processing), real-time APIs, and machine learning models. By adding features such as contextual awareness, API integration, and sentiment analysis, you can develop a highly interactive and efficient bot.
These advanced chatbots can be applied in several fields, including customer support, virtual assistants, and personal companions. The combination of cutting-edge technology and smart features allows them to provide more personalized and relevant responses, enhancing user experience.
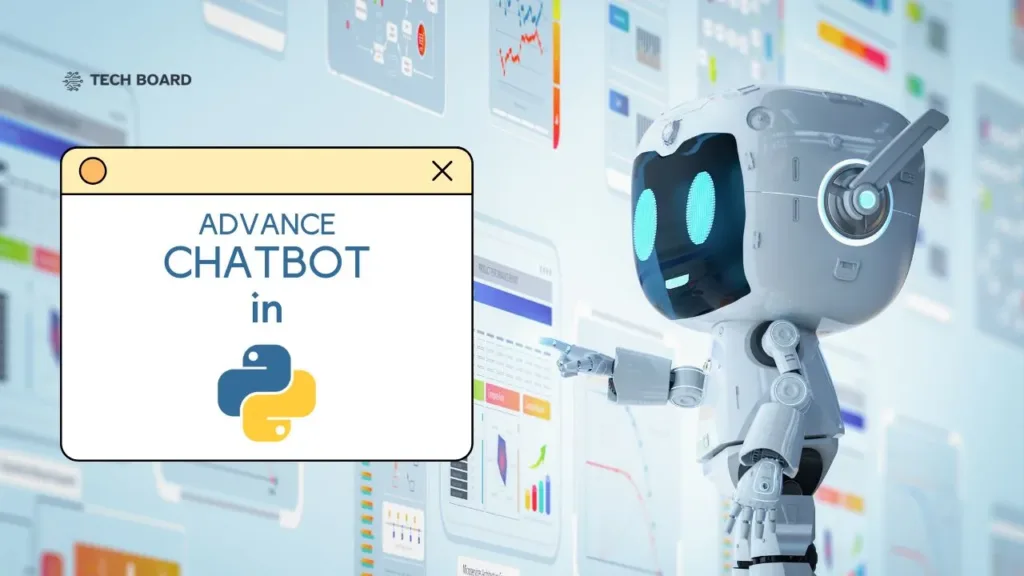
\